Data
Human Api
Free
A better way to build consumer-centric health technology.
# Get all allergies associated with the user
curl -H "Authorization: Bearer someToken" "https://api.humanapi.co/v1/human/medical/allergies"
Coming Soon !
Coming Soon !
Mixpanel
Premium
Simple and powerful analytics that helps everyone make better decisions.
curl --request GET \
--url https://mixpanel.com/api/2.0/insights \
--header 'accept: application/json'
import requests
url = "https://mixpanel.com/api/2.0/insights"
headers = {"accept": "application/json"}
response = requests.get(url, headers=headers)
print(response.text)
const options = {method: 'GET', headers: {accept: 'application/json'}};
fetch('https://mixpanel.com/api/2.0/insights', options)
.then(response => response.json())
.then(response => console.log(response))
.catch(err => console.error(err));
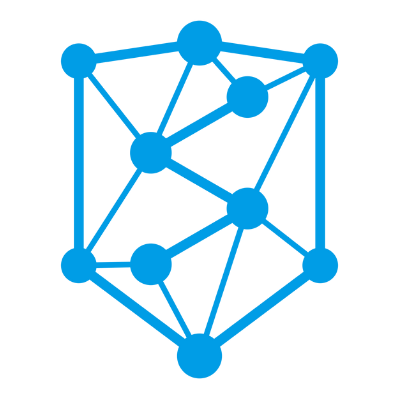
SafeGraph
Free
The most accurate database of global points of interest, curated to power the most innovative applications, platforms, and analytics.
GameSight
Premium
The Gamesight technology platform combines proprietary data, enterprise-grade software, and market expertise to drive revenue for your games.CHAT WITH US
curl --request GET \
--url https://api.marketing.gamesight.io/games \
--header 'X-Api-Version: 2.0.0' \
--header 'accept: application/json'
import requests
url = "https://api.marketing.gamesight.io/games"
headers = {
"accept": "application/json",
"X-Api-Version": "2.0.0"
}
response = requests.get(url, headers=headers)
print(response.text)
const options = {method: 'GET', headers: {accept: 'application/json', 'X-Api-Version': '2.0.0'}};
fetch('https://api.marketing.gamesight.io/games', options)
.then(response => response.json())
.then(response => console.log(response))
.catch(err => console.error(err));

Bloomreach
Free
Find use cases, guides on product integration, API Reference and release notes for each Bloomreach product.
curl --request PATCH \
--url https://api.connect.bloomreach.com/dataconnect/api/v1/accounts/6702/catalogs/catalog_name/products \
--header 'Content-Type: application/json-patch+json' \
--header 'accept: application/json' \
--data '{"op":"add"}'
import requests
url = "https://api.connect.bloomreach.com/dataconnect/api/v1/accounts/6702/catalogs/catalog_name/products"
payload = "{\"op\":\"add\"}"
headers = {
"accept": "application/json",
"Content-Type": "application/json-patch+json"
}
response = requests.patch(url, data=payload, headers=headers)
print(response.text)
const options = {
method: 'PATCH',
headers: {accept: 'application/json', 'Content-Type': 'application/json-patch+json'},
body: '{"op":"add"}'
};
fetch('https://api.connect.bloomreach.com/dataconnect/api/v1/accounts/6702/catalogs/catalog_name/products', options)
.then(response => response.json())
.then(response => console.log(response))
.catch(err => console.error(err));
Opensea
Premium
As the first and largest marketplace for Non-Fungible Tokens and Semi-Fungible Tokens, OpenSea provides a first-in-class developer platform consisting of an API, SDK, and developer tutorials. Feel free to browse around and get acclimated with developing smart contracts and interacting with NFT data.
curl --request GET \
--url 'https://api.opensea.io/v2/collection/slug/nfts?limit=50' \
--header 'accept: application/json'
import requests
url = "https://api.opensea.io/v2/collection/slug/nfts?limit=50"
headers = {"accept": "application/json"}
response = requests.get(url, headers=headers)
print(response.text)
const options = {method: 'GET', headers: {accept: 'application/json'}};
fetch('https://api.opensea.io/v2/collection/slug/nfts?limit=50', options)
.then(response => response.json())
.then(response => console.log(response))
.catch(err => console.error(err));
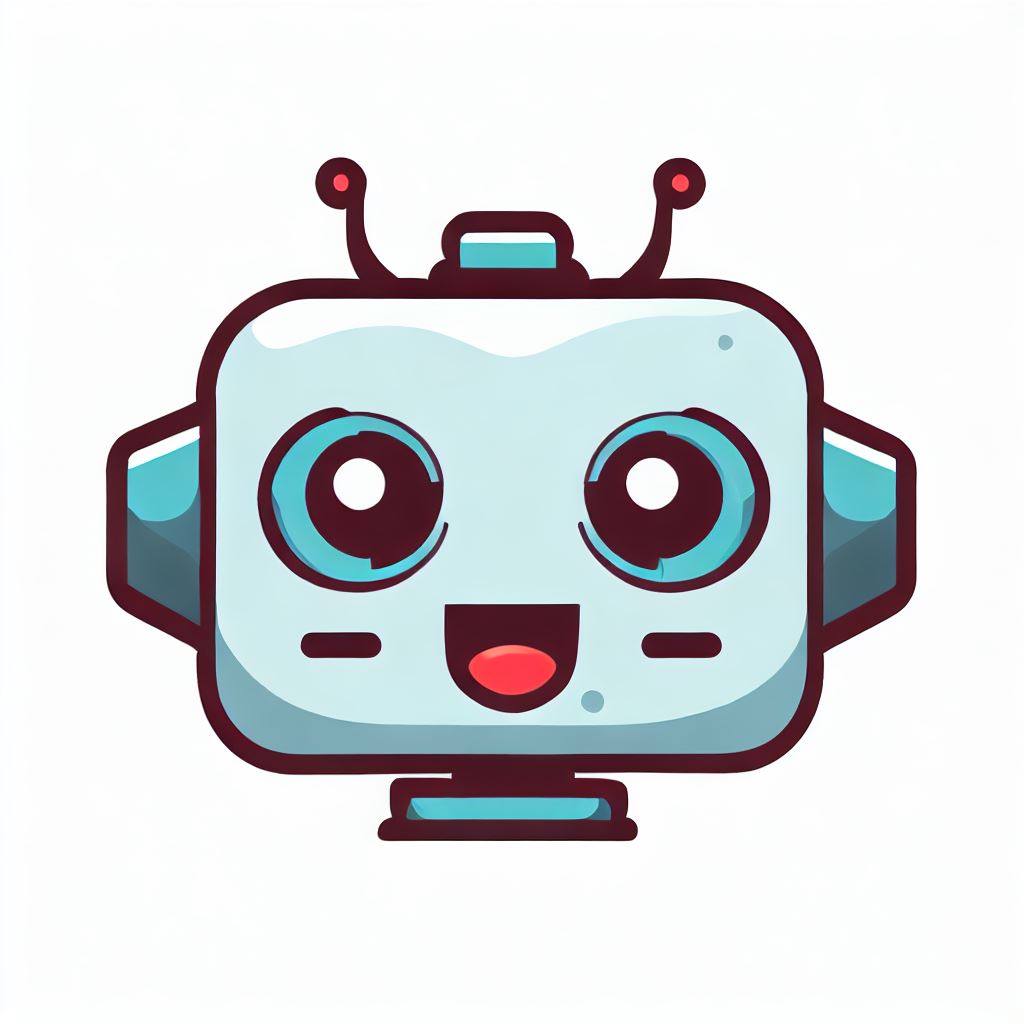